As a web developer, I have come across websites that performed very poorly in terms of loading speed. The main reason being they had too many unoptimized images. Generally, images have to be optimized through resizing and compression to save bandwidth both for the webserver and viewer.
Thanks to advancements in image compression, we now have image formats such as webp which require very less storage space in comparison to the popular formats of yesteryear such as jpeg and png. At the same time, the webp format doesn’t give away with the quality of the image.
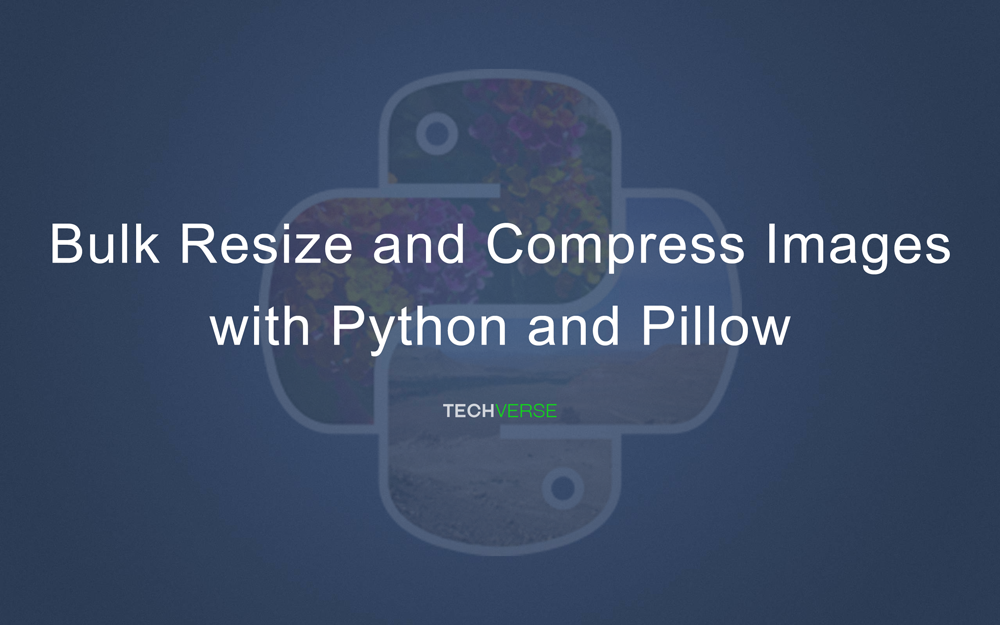
One of my websites was suffering due to unoptimized images. Google’s PageSpeed tool was suggesting me to serve optimized images on the website to decrease the page loading speed. The problem was, this site had more than 20GB of images. It wasn’t going to be an easy job resizing all these jpeg images. After looking around for possible solutions, I zeroed in on python and the Pillow library. Once again python came to my rescue.
Python has this incredibly useful Pillow library for image processing. Pillow supports more than 30 of the most popular image formats available right now. It is a powerful tool for image archiving and batch processing applications. It is commonly used to resize and compress images.
How to Bulk Resize and Compress Images with Python and Pillow
Before we proceed ahead with resizing the images, let’s make sure you have Python 3 installed and then install the Pillow library for python using the following command.
pip install pillow
For this guide, I will be choosing the webp format. You can choose the format according to your requirements. Also don’t forget to create the export path before running the code.
Adjustable variables
In the below code, you can adjust the path, export_path, and fixed_height variable according to your requirements. You can change the output format from webp to any other image format of your choice.
Compression
If you want higher compression, you can reduce the quality value. The quality ranges from 0 to 100. However, I won’t recommend setting the quality too low or the output image won’t look good.
So here’s a small yet powerful piece of code to help you resize and compress images with python.
import PIL
from PIL import Image
import os
path = 'C:/Users/techverse/Desktop/images/'
export_path = 'C:/Users/techverse/Desktop/images/resized/'
images = os.listdir(path)
def resize():
for image in images:
image_name = image.rsplit('.',1)[0]
fixed_height = 250
image = Image.open(path+image)
height_percent = (fixed_height / float(image.size[1]))
width_size = int((float(image.size[0]) * float(height_percent)))
image = image.resize((width_size , fixed_height), PIL.Image.NEAREST)
image.save(export_path+image_name+'.webp', 'webp', optimize=True, quality=90)
resize()
Run the code and once it completes, the images should be stored in the export path. The code works fine both on Windows and Linux (ubuntu). Once the images have been optimized, they will load faster than before.
The code will maintain the aspect ratio of the image. You can change the fixed_height variable according to your requirement. The width of the resized image is set automatically to preserve the aspect ratio.
Here is a screenshot comparing the size of the images before and after resizing and compression.
Before:
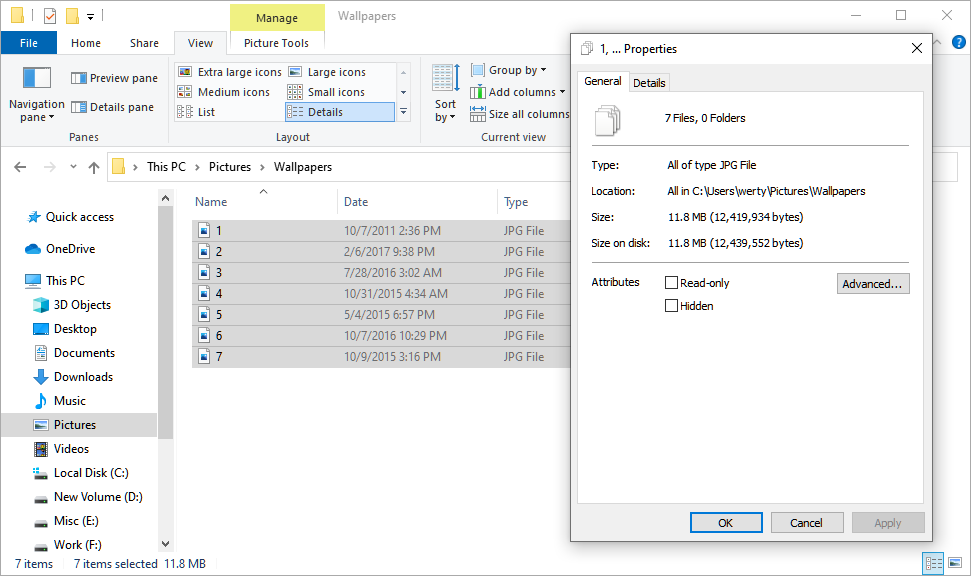
After

From 11.8 MB to 578 KB, the size difference is drastic. Even the quality of the images is nearly identical. As for the 20 GB of images that were compressed using the same code, the total size of the output was around 6 GB.
If you need the output images to have fixed height and width, you can replace the following line in the code.
image = image.resize((width_size , fixed_height), PIL.Image.NEAREST)
with the below code.
image = image.resize((width , height))
With Google taking the mobile-first approach for SEO. The loading speed of a website on mobile devices has become an important ranking factor on google. Since mobile devices don’t have the same processing power as computers, It becomes very important to optimize all bandwidth-heavy elements such as images to increase the loading speed. As of today, a faster loading speed on mobile devices means a better ranking for your website on Google.
While my use for resizing and compressing images with python and pillow was focused on reducing the loading speed of websites. You can use the above code for batch resizing and compressing images for any other application.
Really awesome article. Thanks for sharing that type of good content.
Keep it up with good content.