Those coming from WordPress will be very much familiar with the term slug. For those who are new, Slug is the part of the URL that comes after the domain name. The slug provides a unique identity to any page on a website. On content management systems like WordPress, the slug is automatically generated from the title.
Slugs play an important role in search engine optimization. A well-optimized slug is usually short and contains the primary keyword of the post. Search engines prefer pretty URLs which are made of text separated by a dash. This URL structure is preferred as it’s easily understandable by the user as well as search engines in comparison to having parameters in the URL.
Laravel does not come with any SEO feature inbuilt. It’s a web application framework, so it doesn’t need to have one. Most of the on-page SEO can be done by web developers by using proper HTML tags. However, for creating slugs, we can make use of one of laravel’s helper methods.
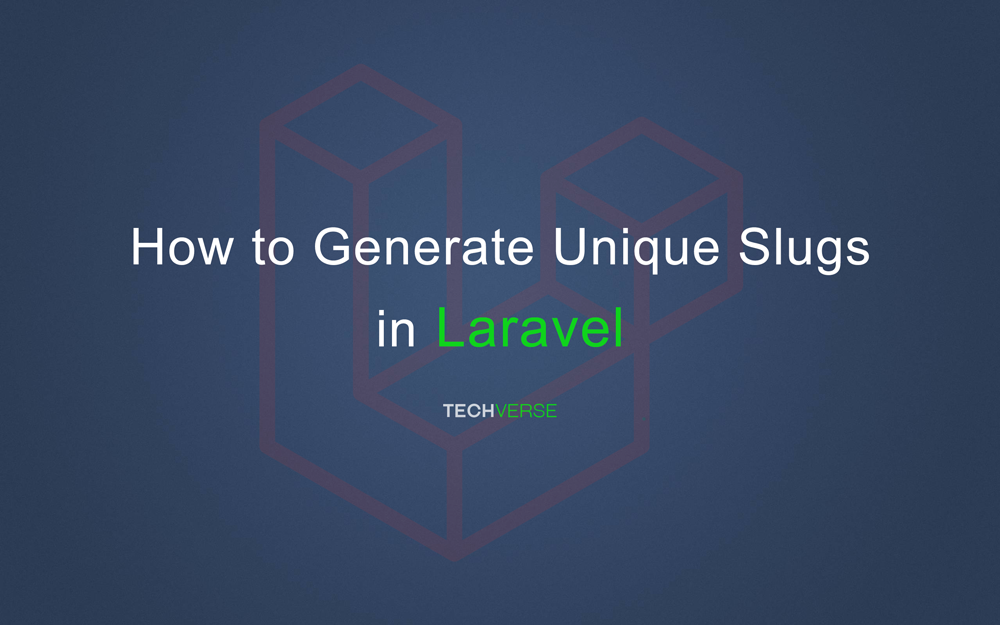
How to Generate Unique Slug in Laravel
The Str helper class has a slug method that can be used to generate slugs from text. As usual, before we can use the Str::slug() helper method, we will need to import the class into the controller.
use Illuminate\Support\Str;
After that, we can pass on a text into the Str::slug method to generate a slug out of the text. Here’s an example of the same.
$slug = Str::slug('How to Generate Unique Slugs in Laravel', '-');
The above code will generate the following slug.
how-to-generate-unique-slugs-in-laravel
This looks much better than using the id of the post as the slug. You can save the slug in the database with a unique index. Then use it instead of the id to fetch data for the post.
That’s how you can easily generate SEO-friendly URLs in laravel. Continue reading to add uniqueness to the slug.
Make Slugs Truly Unique
There’s one more thing you need to do to make a truly unique slug. There is a probability that in the future you might create another post with the same title. At that point, you will face an error as the database won’t allow storing two exactly same slugs on a column with the unique index.
In order to eliminate any chances of having that error, we will take an extra step to ensure we never have to worry about duplicate slugs again. For this, we will create another column with a unique hash for the post. You can use the below function to generate random alphanumeric codes of any length. The generated codes will not be truly unique, but the combination of the slug and the hash will create a unique slug.
public function random_strings($length_of_string) {
$str_result = '0123456789abcdefghijklmnopqrstuvwxyz';
return substr(str_shuffle($str_result), 0, $length_of_string);
}
You can call the above function like this:
$hash = $this->random_strings(6);
So the final slug will be a combination of the randomly generated hash and the slug of the title:
hash + slug
Cy8urt-how-to-generate-unique-slugs-in-laravel
Now when you have to retrieve data for the post, you can split the URL at the first dash and separate the hash and slug. The slug and hash can then be used to retrieve data for the page. This can be achieved with the explode function in PHP.
$split = explode('-', 'Cy8urt-how-to-generate-unique-slugs-in-laravel',2);
$hash = $split[0];
// Cy8urt
$slug = $split[1];
// how-to-generate-unique-slugs-in-laravel
These variables can be then used to query data for the page.
$post = Post::where( ['slug', '=', $slug, ‘hash’, '=', $hash] )->firstOrFail();
With this, the URLs generated by the laravel app will always be unique. While this was my approach to creating a unique slug in laravel, do let me know if you have a better approach in your mind.