Laravel is built with security in mind and hence it comes with a lot of built-in security features. One of these major security features is cross-site request forgery (CSRF) protection. The csrf vulnerability allows anyone to imitate forms on a site and make forged requests to modify or retrieve data.
Laravel generates a csrf token for every user session. Using the csrf token, laravel can distinguish between requests made by authenticated users and malicious ones. This allows laravel to block malicious requests not origination from its users.
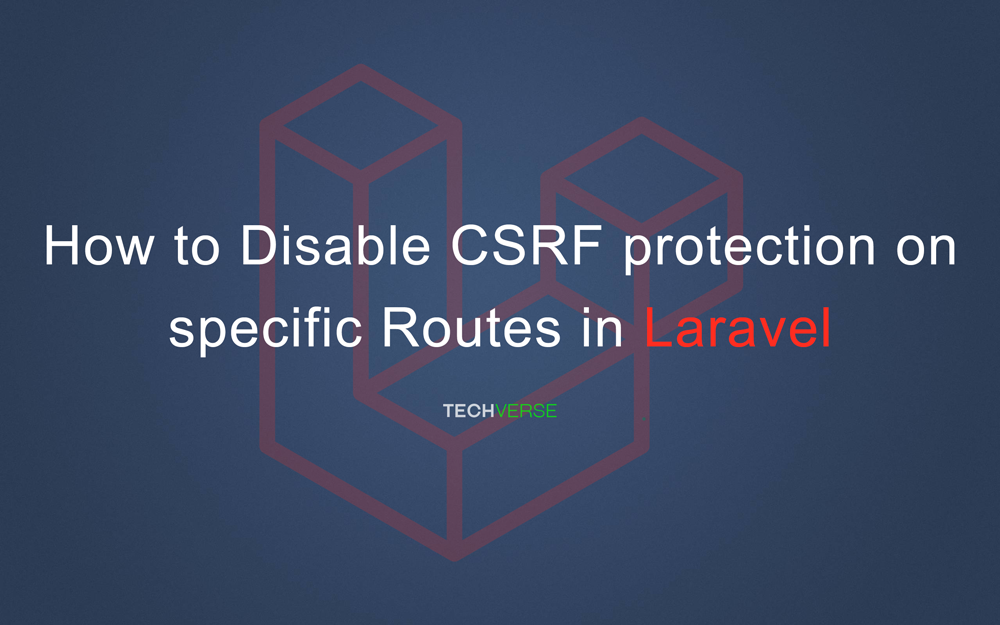
If you have implemented an HTML form in laravel, you might remember adding the @csrf directive. without the csrf directive, the form would have refused to work. The @csrf directive automatically generates a hidden input field. The input field has the csrf token generated by laravel as the value. This ensures the csrf token is always included with requests from any form.
While csrf protection is there to provide security to the user, there are certain situations when the csrf protection needs to be disabled for some features to work.
For example, I implemented an API in one of my projects. The API received processed data from an external script via a post request. This is where laravel’s csrf protection becomes an obstacle. With csrf protection enabled, all post requests to the API endpoint from the external script were being blocked as they had no csrf token.
My only option was to disable csrf protection on the specific API route. Without this, the external script would not have been able to post data to the laravel app. Luckily, the information for the same was available in laravel’s documentation.
How to Disable CSRF protection on specific Routes in Laravel
To disable csrf protection on specific routes, the $except property of the VerifyCsrfToken middleware has to be updated with the URI or routes which need to be excluded from csrf protection.
The file can be found in the \var\www\laravel\app\Http\Middleware folder in ubuntu. The following code is an example with the updated $except property containing the excluded routes.
<?php
namespace App\Http\Middleware;
use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware;
class VerifyCsrfToken extends Middleware
{
/**
* The URIs that should be excluded from CSRF verification.
*
* @var array
*/
protected $except = [
'api/*',
'http://example.com/api/*',
'http://example.com/api/receive',
];
}
You can add both web and APi routes to the $except property. With this simple change, you can exclude specific routes in laravel from csrf protection.
However, with csrf protection disabled on the routes, make sure you include other verification methods such as including a secret key with each request, where the secret key is only known to the app and the script. This will stop anyone without the knowledge of the secret key to access the API.